Q: 1 - Q.30 carry one m ark each
1. The goal of structured programming is to
(a) have well indented programs
(b) be able to infer the flow of control from the compiled code
(c) be able to infer the flow of control form the program text
(d) avoid the use of GOTO statements
2. Consider the following C function
vaid swap (int a, int b)
{ int temp;
temp = a ;
a = b ;
b= temp ;
}
In order to exchange the values of two variables x and y.
(a) call swap (x, y)
(b) call swap (&x, &y)
(c) swap (x,y) cannot be used as it does not return any value
(d) swap (x,y) cannot be used as the parameters are passed by value
3. A single array A[1..MAXSIZE] is used to implement two stacks. The two stacks grow from opposite ends of the array. Variables top1 and top 2 (top1< top 2) point to the location of the topmost element in each of the stacks. If the space is to be used efficiently, the condition for "stack full" is
(a) (top1 = MAXSIZE/2) and (top2 = MAXSIZE/2+1)
(b) top1 + top2 = MAXSIZE
(c) (top1 = MAXSIZE/2) or (top2 = MAXSIZE)
(d) top1 = top2 -1
4. The following numbers are inserted into an empty binary search tree in the given order: 10, 1, 3, 5, 15, 12, 16. What is the height of the binary search tree (the height is the maximum distance of a leaf node from the root)?
(a) 2 (b) 3 (c) 4 (d) 6
5. The best data structure to check whether an arithmetic expression has balanced parentheses is a
(a) queue (b) stack (c) tree (d) list
6. Level order traversal of a rooted tree can be done by starting from the root and performing
(a) preorder traversal (b) in-order traversal
(c) depth first search (d) breadth first search
7. Given the following input (4322, 1334, 1471, 9679, 1989, 6171, 6173, 4199) and the hash function x mod 10, which of the following statements are true?
i) 9679, 1989, 4199 hash to the same value
ii) 1471, 6171 has to the same value
iii) All elements hash to the same value
iv) Each element hashes to a different value
(a) i only (b) ii only (c) i and ii only (d) iii or iv
8. Which of the following grammar rules violate the requirements of an operator grammar? P, Q, R are nonterminals, and r,s,t are terminals.
(i) P ® Q R (ii) P ® Q s R (iii) P ® e (iv) P ® Q t R r
(a) (i) only (b) (i) and (iii) only
(c) (ii) and (iii) only (d) (iii) and (iv) only
9. Consider a program P that consists of two source modules M1 and M2 contained in two different files. If M1 contains a reference to a function defined in M2 the reference will be resolved at
(a) Edit time (b) Compile time (c) Link time (d) Load time
10. Consider the grammar rule E ® E1 - E2 for arithmetic expressions. The code generated is targeted to a CPU having a single user register. The subtraction operation requires the first operand to be in the register. If E1 and E2 do not have nay-common sub-expression, in order to get the shortest possible code
(a) E1 should be evaluated first
(b) E2 should be evaluated first
(c) Evaluation of E1 and E2 should necessarily be interleaved
(d) Order to evaluation of E1 and E2 is of no consequence
11. Consider the following statements with respect to user-level threads and kernel-supported threads
(i) context switch is faster with kernel-supported threads
(ii) for user-level threads, a system call can block the entire process
(iii) Kernel supported threads can be scheduled independently
(iv) User level threads are transparent to the kernel
Which of the above statements are true?
(a) (ii), (iii) and (iv) only (b) (ii) and (iii) only
(c) (i) and (iii) only (d) (i) and (ii) only
12. Consider an operating system capable of loading and executing a single sequential user process at a time. The disk head scheduling algorithm used is First Come First Served (FCFS). If FCFS is replaced by Shortest Seek Time First (SSTF), claimed by the vendor to give 50% better benchmark results, what is the expected improvement in the I/O performance of user programs?
(a) 50% (b) 40% (c) 25% (d) 0%
13. Let R1 (
, B, ((D) and R2 (
, E) be two relation schema, where the primary keys are shown underlined, and let C be a foreign key in R1 referring to R2 . Suppose there is no violation of the above referential integrity constraint in the corresponding relation instances r1and r2 . Which one of the following relational algebra expressions would necessarily produce an empty relation?


(a) ÕD (r2) - ÕC (r1) (b) ÕC (r1) - ÕD (r2)
(c) ÕD (r1
C ¹D r2) (d) ÕC (r1
C =D r2)


14. Consider the following relation schema pertaining to a students database:
Students (rollno, name, address)
Enroll(rollno,courseno, coursename)
Where the primary keys are shown underlined. The number of tuples in the student and Enroll tables are 120 and 8 respectively. What are the maximum and minimum number of tuples that can be present in (Student * Enroll), where '*' denotes natural join?
(a) 8, 8 (b) 120, 8 (c) 960, 8 (d) 960, 120
15. Choose the best matching between Group 1 and Group 2
Group -1 Group - 2
P. Data link layer 1. Ensures reliable transport of data over a physical point-to-point link
Q. Network layer 2. Encodes/decodes data for physical transmission
R. Transport layer 3. Allows end-to-end communication between two processes
4. Routes data from one network node to the next
(a) P - 1, Q - 4, R - 3 (b) P - 2, Q - 4, R - 1
(c) P - 2, Q - 3, R - 1 (d) P - 1, Q - 3, R - 2
16. Which of the following is NOT true with respect to a transparent bridge and a router?
(a) Both bridge and router selectively forward data packets
(b) A bridge uses IP addresses while a router uses MAC addresses
(c) A bridge builds up its routing table by inspecting incoming packets
(d) A router can connect between a LAN and a WAN
17. A Boolean function x¢y¢ + xy + x¢y is equivalent to
(a) x¢ + y¢ (b) x + y (c) x + y¢ (d) x¢ + y¢
18. In an SR latch made by cross-coupling two NAND gates, if both S and R inputs are set to 0, then it will result in
(a) Q = 0, Q¢ = 1 (b) Q = 1, Q¢ = 0
(c) Q = 1, Q¢ = 1 (d) Indeterminate states
19. If 73x (in base-x number system) is equal to 54y (in base y-number system), the possible values of x and y are
(a) 8, 16 (b) 10, 12 (c) 9, 13 (d) 8, 11
20. Which of the following addressing modes are suitable for program relocation at run time?
(i) Absolute addressing (ii) Based addressing
(iii) Relative addressing (iv) Indirect addressing
(a) (i) and (iv) (b) (i) and (ii) (c) (ii) and (iii) (d) (i), (ii) and (iv)
21. The minimum number of page frames that must be allocated to a running process in a virtual memory environment is determined by
(a) the instruction set architecture (b) page size
(c) physical memory size (d) number of processes in memory
22. How many 8-bit characters can be transmitted per second over a 9600 baud serial communication link using asynchronous mode of transmission with one start bit, eight data bits, two stop bits, and one parity bit?
(a) 600 (b) 800 (c) 876 (d) 1200
23. Identify the correct translation into logical notation of the following assertion. Some boys in the class are taller than all the girls
Note: taller (x, y) is true if x is taller than y.
(a) ($x) (boy(x) ® ("y) (girl(y) Ù taller (x, y)))
(b) ($x) (boy(x) Ù ("y) (girl(y) Ù taller (x, y)))
(c) ($x) (boy(x) ® ("y) (girl(y) ® taller (x, y)))
(d) ($x) (boy(x) Ù ("y) (girl(y) Ù taller (x, y)))
24. Consider the binary relation:
S = {(x, y)|y = x + 1 and x, y Î {0, 1, 2}}
The reflexive transitive closure of S is
(a) {(x, y)|y > x and x, y Î {0, 1, 2}} (b) {(x, y)|y ³ x and x, y Î {0, 1, 2}}
(c) {(x, y)|y < x and x, y Î {0, 1, 2}} (b) {(x, y)|y £ x and x, y Î {0, 1, 2}}
25. If a fair coin is tossed four times. What is the probability that two heads and two tails will result?
(a)
(b)
(c)
(d) 




26. The number of different n × n symmetric matrices with each element being either 0 or 1 is : (Note: power (2,x) is same as 2x)
(a) power (2,n) (b) power(2,n2)
(c) power (2, (n2 +n/2) (d) power (2, (n2 - n)/2)
27. Let A,B,C,D be n × n matrices, each with non-zero determinant. If ABCD=I, then B-1 is
(a) D-1 C-1 A-1 (b) CDA
(c) ADC (d) Does not necessarily exist
28. What is the result of evaluating the following two expressions using three-digit floating point arithmetic with rounding?
(113. + - 111.) + 7.51
113. + (- 111.) + 7.51)
(a) 9.51 and 10.0 respectively (b) 10.0 and 9.51 respectively
(c) 9.51 and 9.51 respectively (d) 10.0 and 10.0 respectively
29. The tightest lower bound on the number of comparisons, in the worst case, for comparison-based sorting is of the order of
(a) n (b) n2 (c) nlogn (d) nlog2n
30. The problem 3-SAT and 2-SAT are
(a) both in P
(b) both NP complete
(c) NP-complete and in P respectively
(d) undecidable and NP-complete respectively
Q: 31 - 90 carry two marks each
31. Consider the following C function:
int f(int n)
{ static int i = 1 ;
if (n >=5) return n;
n = n+I;
i++;
return f(n);
}
The value returned by f(1) is
(a) 5 (b) 6 (c) 7 (d) 8
32. Consider the following program fragment for reversing the digits in a given integer to obtain a new integer. Let n = d1 d2 …dm .
int n, rev;
rev = 0;
while (n > 0) {
rev = rev *10+n % 10;
n = n / 10 ;
}
The loop invariant condition at the end of the i iteration is: th
(a) n = d1d2...dm-1and rev=dmdm-1...dm-1+1
(b) n = dm+1...dm-1dm or rev= dm-1...d2d1
(c) n ¹ rev
(d) n = d1d2... 1dm or rev =dm...d2d1
33. Consider the following C program segment:
char p [20]
char * s = "string";
int length = strlen (s);
for (i = 0 ; i < length; i++)
p[i] = s[length - I];
print f("%",p);
The output of the program is
(a) gnirts (b) string
(c) gnirt (d) no output is printed
34. It is desired to design an object-oriented employee record system for a company. Each employee has a name, unique id and salary. Employees belong to different categories and their salary is etermined by their category. The functions get Name, getld and compute salary are required. Given the class hierarchy below,possible locations for these functions are:
(i) getld is implemented in the superclass
(ii) getld is implemented in the subclass
(iii) getName is an abstract function in the superclass
(iv) getName is implemented in the superclass
(v) getName is implemented in the subclass
(vi) getSalary is an abstract function in the superclass
(vii) getSalary is implemented in the superclass
(viii) getSalary is implemented in the subclass
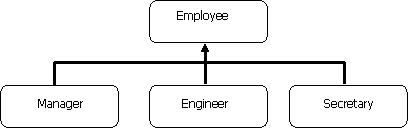
Choose the best design
(a) (i), (iv), (vi), (viii) (b) (i), (iv), (vii)
(c) (i), (iii), (v), (vi), (viii) (d) (ii), (v), (viii)
35. Consider the label sequences obtained by the following pairs of traversals on a labeled binary tree. Which of these pairs identify a tree uniquely?
(i) preorder and postorder (ii) inorder and postorder
(iii) preorder and inorder (iv) level order and postorder
(a) (i) only (b) (ii), (iii) (c) (iii) only (d) (iv) only
36. A circularly linked list is used to represent a Queue. A single variable p is used to access the Queue. To which node should p point such that both the operations enQueue and deQueue can be performed in constant time?
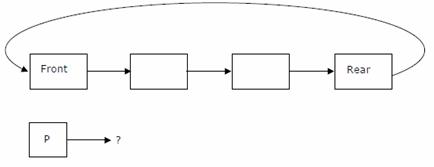
(a) rear node (b) front node
(c) not possible with a single pointer (d) node next to front
37. The elements 32, 15, 20, 30, 12, 25, 16, are inserted one by one in the iven order into a maxHeap. The resultant maxHeap is
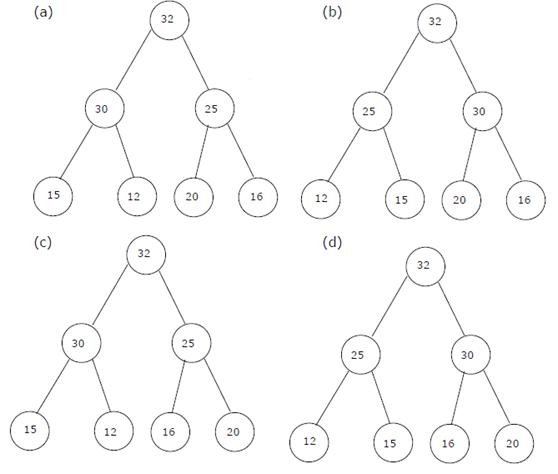
38. Assume that the operators +, -, × , are left associative and ^ is right associative. the order of precedence (from highest to lowest) is ^, × , +, -. The postfix expression corresponding to the infix expression a + b × c-d^e^f is
(a) abc×+def^^- (b) abc×+de^f^-
(c) ab+c×d-e^f^ (d) - + a×bc^^def
39. Two matrices M1 and M2 are to be stored in arrays A and B respectively. Each array can be stored either in row-major or column-major order in contiguous memory locations. The time complexity of an algorithm to compute M1 × M2 will be
(a) best if A is in row-major, and B is in column major order
(b) best if both are in row-major order
(c) best if both are in column-major order
(d) independent of the storage scheme
40. Suppose each set is represented as a linked list with elements in arbitray order. Which of the operations among union, intersection, membership, cardinality will be the slowest?
(a) union only (b) intersection, membership
(c) membership, cardinality (d) union, intersection
41. Consider the following C program
main ( )
{ int x, y, m, n;
scanf ("%d %d", &x, &y);
/ *Assume x > 0 and y > 0 */
m = x; n = y;
while (m! = n)
{ if (m > n)
m = m - n;
else
n = n - m;
}
printf("%d",n);
}
The program computes
(a) x + y using repeated subtraction
(b) x mod y using repeated subtraction
(c) the greatest common divisor of x and y
(d) the least common multiple of x and y
42. What does the following algorithm approximate? (Assume m > 1,Î > 0).
X = m;
Y = 1;
While (x - y > Î)
{ x = (x + y) / 2;
y = m/x;
}
print (x);
(a) log m (b) m2 (c) m1/2 (d) m1/3
43. Consider the following C program segment
struct CellNode{
struct CellNode *leftChild;
int element;
struct CellNode *rightChild;
};
int Dosomething (struct CellNode *ptr)
{
int value = 0;
if (ptr ! = NULL)
{ if (ptr - > leftChild ! = NULL)
value = 1 + DoSomething (ptr - > leftChild);
if (ptr - > rightChild ! = NULL)
value = max(value,1 + DoSomething (ptr - > rightChild));
}
return (value);
}
The value returned by the function DoSomething when a pointer to the root of a non-empty tree is passed as argument is
(a) The number of leaf nodes in the tree
(b) The number of nodes in the tree
(c) The number of internal nodes in the tree
(d) The height of the tree
44. Suppose we run Dijkstra's single source shortest-path algorithm on the following edge-weighted directed graph with vertex P as the source.
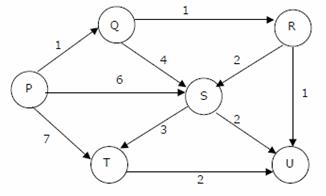
In what order do the nodes get included into the set of vertices for which the shortest path distances are finalized?
(a) P,Q,R,S,T,U (b) P,Q,R,U,S,T
(c) P,Q,R,U,T,S (d) P,Q,T,R,U,S
45. Consider the grammar with the following translation rules and E as the start symbol.
E® E1 # T {E.value = E1.value * T.value}
| T {E.value = T.value}
T ® T1 & F {T.value = T1 .value * F.value}
| F {T.value = F.value}
F® num {F.value = num.value}
Compute E.value for the root of the parse tree for the expression:2 # 3 & 5 # 6 & 4.
(a) 200 (b) 180 (c) 160 (d) 40
46. Consider the following set of processes, with the arrival times and the CPU-burst times given in milliseconds.
Process Arrival Time Burst Time
P1 0 5
P2 1 3
P3 2 3
P4 4 1
What is the average turnaround time for these processes with the preemptive shortest remaining processing time first (SRPT) algorithm?
(a) 5.50 (b) 5.75 (c) 6.00 (d) 6.25
47. Consider a system with a two-level paging scheme in which a regular memory access takes 150 nanoseconds, and servicing a page fault takes 8 milliseconds. An average instruction takes 100 nanoseconds of CPU time, and two memory accesses. The TLB hit ratio is 90%, and the page fault rate is one in every 10,000 instructions. What is the effective average instruction execution time?
(a) 645 nanoseconds (b) 1050 nanoseconds
(c) 1215 nanoseconds (d) 1230 nanoseconds
48. Consider two processes P1 and P2 accessing the shared variables X and Y protected by two binary semaphores Sx and Sy respectively, both initialized to 1.P and V denote the usual semaphore operators, where P decrements the semaphore value, and V increments the semaphore value. The pseudo-code of P1 and P2 is as follows:
p1: p2:
While true do { While true do {
L1;................ L3...............
L2;................ L4;................
X = X + 1; Y = Y + 1;
Y = Y - 1; X = Y - 1;
V(Sx); V(Sy);
V(Sy); V(Sx);
In order to avoid deadlock, the correct operators at L1 , L2 , L3 and L4 are respectively.
(a) p (sy), p (sx); p (sx), p(sy) (b) p (sx), p(sy) ; p (sy), p (sx)
(c) p (sx), p (sx); p (sy), p (sy) (d) p (sy), p (sy); p (sx), p (sy);
49. A unix-style I-node has 10 direct pointers and one single, one double and one triple indirect pointers. Disk block size is 1 Kbyte, disk block address is 32 bits, and 48-bit integers are used. What is the maximum possible file size?
(a) 224 bytes (b) 232 bytes (c) 234 bytes (d) 248 bytes
50. The relation scheme Student Performance (name, courseNo, rollNo, grade) has the following functional dependencies:
name, courseNo, ® grade
rollNo, courseNo ® grade
name ® rollNo
rollNo ® name
The highest normal form of this relation scheme is
(a) 2 NF (b) 3 NF (c) BCNF (d) 4 NF
51. Consider the relation Student (name, sex, marks), where the primary key is shown underlined, pertaining to students in a class that has at least one boy and one girl. What does the following relational algebra expression produce)
(Note: r is the rename operator).
Õname (rsex=female (Student)) - Õname 

(a) names of girl students with the highest marks
(b) names of girl students with more marks than some boy student
(c) names of girl students with marks not less than some boy student
(d) names of girl students with more marks than all the boy students
52. The order of an internal node in a B+ tree index is the maximum number of children it can have. Suppose that a child pointer takes 6 bytes, the search field value takes 14 bytes, and the block size is 512 bytes. What is the order of the internal node?
(a) 24 (b) 25 (c) 26 (d) 27
53. The employee information in a company is stored in the relation
Employee (name, sex, salary, deptName)
Consider the following SQL query
Select deptName
From Employee
where sex = "M'
Group by deptName
Having avg(salary) >
(select avg (salary) from Employee)
It returns the names of the department in which
(a) the average salary is more than the average salary in the company
(b) the average salary of male employees is more than the average salary of all male employees in the company
(c) the average salary of male employees is more than the average salary of employees in the same department.
(d) the average salary of male employees is more than the average salary in the company
54. A and B are the only two stations on an Ethernet. Each has a steady queue of frames to send. Both A and B attempt to transmit a frame, collide, and A wins the first backoff race. At the end of this successful transmission by A, both A and B attempt to transmit and collide. The probability that A wins the second backoff race is
(a) 0.5 (b) 0.625 (c) 0.75 (d) 1.0
55. The routing table of a router is shown below:
Destination Subnet Mask Interface
128.75.43.0 255.255.255.0 Eth0
128.75.43.0 255.255.255.128 Eth1
192.12.17.5 255.255.255.255 Eth3
Default Eth2
On which interface will the router forward packets addressed to destinations 128.75.43.16 and 192.12.17.10 respectively?
(a) Eth1 and Eth2 (b) Eth0 and Eth2
(c) Eth0 and Eth3 (d) Eth1 and Eth3
The following information pertains to Q.56 and 57:
Consider three IP networks A, B and C. Host HA in networks A sends messages each containing 180 bytes of application data to a host HC in network C. The TCP layer prefixes a 20 byte header to the message. This passes through an intermediate network B. the maximum packet size, including 20 byte IP header, in each network is:
A : 1000 bytes
B : 100 bytes
C : 1000 bytes
The network A and B are connected through a 1 Mbps link, while B and C are connected by a 512 Kbps link (bps = bits per second).

56. Assuming that the packets are correctly delivered, how many bytes, including headers, are delivered to the IP layer at the destination for one application message, in the best case? Consider only data packets.
(a) 200 (b) 220 (c) 240 (d) 260
57. What is the rate at which application data is transferred to host HC? Ignore errors, acknowledgements, and other overheads.
(a) 325.5 Kbps (b) 354.5 Kbps (c) 409.6 Kbps (d) 512.0 Kbps
58. A circuit outputs a digit in the form of 4 bits. 0 is represented by 0000, 1 by 0001, …, 9 by 1001. A combinational circuit is to be designed which takes these 4 bits as input and outputs 1 if the digit ³ 5, and 0 otherwise. If only AND, OR and NOT gates may be used, what is the minimum number of gates required?
(a) 2 (b) 3 (c) 4 (d) 5
59. Which are the essential prime implicants of the following Boolean function?
f(a, b, c) = a¢c + ac¢ + b¢c
(a) a¢c and ac¢ (b) a¢c and b¢c (c) a¢c only (d) ac¢ and bc¢
60. Consider a multiplexer with X and Y as data inputs and Z as control input. Z = 0 selects input X, and Z = 1 selects input Y. What are the connections required to realize the 2-variable Boolean function f = T + R, without using any additional hardware?
(a) R to X, 1 to Y, T to Z (b) T to X, R to Y, T to Z
(c) T to X, R to Y, 0 to Z (d) R to X, 0 to Y, T to Z
61. Consider the partial implementation of a 2-bit counter using T flip-flops following the sequence 0-2-3-1-0, as shown below.
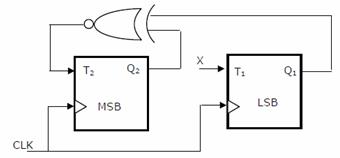
To complete the circuit, the input X should be
(a)
(b) Q2 + Q1 (c) (Q1 Å Q2)¢ (d) Q1 Å Q2

62. A 4-bit carry look ahead adder, which adds two 4-bit numbers, is designed using AND, OR, NOT, NAND, NOR gates only. Assuming that all the inputs are available in both complemented and uncomplemented forms and the delay of each gate is one time unit, what is the overall propagation delay of the adder? Assume that the carry network has been implemented using two-level AND-OR logic.
(a) 4 time units (b) 6 time units (c) 10 time units (d) 12 time units
The following information pertains to Q.63 and 64:
Consider the following program segment for a hypothetical CPU having three user registers R1, R2 and R3.
Instruction Operation Instruction Size (in words)
MOV R1,5000 ;R1 ¬ Memory[5000] 2
MOV R2(R1) ;R2 ¬ Memory[(R1)] 1
ADD R2, R3 ;R2 ¬ R2 + R3 1
MOV 6000, R2 ; Memory[6000] ¬ R2 2
HALT ;Machine halts 1
63. Consider that the memory is byte addressable with size 32 bits, and the program has been loaded starting form memory location 1000 (decimal). If an interrupt occurs while the CPU has been halted after executing the HALT instruction, the return address (in decimal) saved in the stack will be
(a) 1007 (b) 1020 (c) 1024 (d) 1028
64. Let the clock cycles required fro various operations be as follows:
Register to/from memory transfer : 3 clock cycles
ADD with both operands in register : 1 clock cycle
Instruction fetch and decode : 2 clock cycles per word
The total number of clock cycles required to execute the program is
(a) 29 (b) 24 (c) 23 (d) 20
65. Consider a small two-way set-associative cache memory, consisting of four blocks. For choosing the block to be replaced, use the least recently used (LRU) scheme. The number of cache misses for the following sequence of block addresses is 8, 12, 0, 12,8
(a) 2 (b) 3 (c) 4 (d) 5
66. Let A = 1111 1010 and B = 0000 1010 be two 8-bit 2's complement numbers.
Their product in 2's complement is
(a) 1100 0100 (b) 1001 1100 (c) 1010 0101 (d) 1101 0101
67. The microinstructions stored in the control memory of a processor have a width of 26 bits. Each microinstruction is divided into three fields: a micro-operation field of 13 bits, a next address field (X), and a MUX select field (Y). There are 8 status bits in the inputs of the MUX. How many bits are there in the X and Y fields, and what is the size of the control memory in number of words?
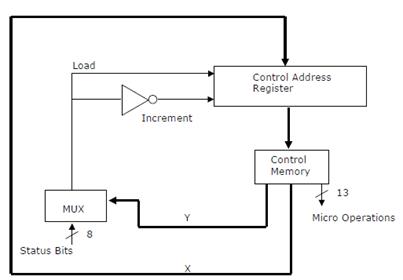
(a) 10, 3, 1024
(b) 8, 5, 256
(c) 5, 8, 2048
(d) 10, 3, 512
68. A hard disk with a transfer rate of 10 Mbytes/second is constantly transferring data to memory using DMA. The processor runs at 600 MHz, and takes 300 and 900 clock cycles to initiate and complete DMA transfer respectively. If the size of the transfer is 20 Kbytes, what is the percentage of processor time consumed for the transfer operation?
(a) 5.0% (b) 1.0% (c) 0.5% (d) 0.1%
69. A 4-stage pipeline has the stage delays as 150, 120, 160 and 140 nanoseconds respectively. Registers that are used between the stages have a delay of 5 nanoseconds each. Assuming constant clocking rate, the total time taken to process 1000 data items on this pipeline will be
(a) 120.4 microseconds (b) 160.5 microseconds
(c) 165.5 microseconds (d) 590.0 microseconds
70. The following prepositional statement is (P ® (Q Ú R)) ® ((P Ù Q) ® R
(a) satisfiable but not valid (b) valid
(c) a contradiction (d) None of the above
71. How many solutions does the following system of linear equations have?
-x + 5y = -1
x - y = 2
x + 3y = 3
(a) infinitely many (b) two distinct solutions
(c) unique (d) none of the above
72. The following is the incomplete operation table of a 4-element group.
*
|
e
|
a
|
b
|
c
|
e
|
e
|
a
|
b
|
c
|
a
|
a
|
b
|
c
|
e
|
b
| ||||
c
|
The last row of the table is
(a) c a e b (b) c b a e (c) c b e a (d) c e a b
73. The inclusion of which of the following sets into
S = {{1, 2}, {1, 2, 3}, {1, 3, 5}, {1, 2, 4}, {1, 2, 3, 4, 5}}
is necessary and sufficient to make S a complete lattice under the partial order defined by set containment?
(a) {1} (b) {1}, {2, 3}
(c) {1}, {1, 3} (d) {1}, {1, 3}, {1, 2, 3, 4}, {1, 2, 3, 5}
74. An examination paper has 150 multiple choice questions of one mark each, with each question having four choices. Each incorrect answer fetches -0.25 marks. Suppose 1000 students choose all their answers randomly with uniform probability. The sum total of the expected marks obtained by all these students is
(a) 0 (b) 2550 (c) 7525 (d) 9375
75. Mala has a colouring book in which each English letter is drawn two times. She wants to paint each of these 52 prints with one of k colours, such that he colour pairs used to colour any two lwtters are different. Both prints of a letter can also be coloured with the same colour. What is the minimum value of k that satisfies this requirement?
(a) 9 (b) 8 (c) 7 (d) 6
76. In an M ´ N matrix such that all non-zero entries are covered in a rows and b columns. Then the maximum number of non-zero entries, such that no two are on the same row or column, is
(a) £ a + b (b) £ max(a, b)
(c) £ min(M - a, N - b) (d) £ min(a, b)
77. The minimum number of colours required to colour the following graph, such that no two adjacent vertices are assigned the same colour, is
![]() |
(a) 2 (b) 3 (c) 4 (d) 5
78. Two n bit binary strings, S1 and S2 are chosen randomly with uniform probability.The probability that the Hamming distance between these strings (the number of it positions where the two strings differ) is equal to d is
(a) nCd / 2n (b) nCd / 2d (c) d / 2n (d) 1 / 2d
79. How many graph on n labeled vertices exist which have at least
edges.

(a)
(b) 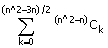
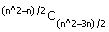
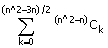
(c)
(d)


80. A point is randomly selected with uniform probability in the X-Y plane within the rectangle with corners at (0,0), (1,0), (1,2) and (0,2). If p is the length of the position vector of the point, the expected value of p2 is
(a)
(b) 1 (c)
(d) 



81. Let G1 = (V , E1) and G2 = (V , E2) be connected graphs on the same vertex set V , with more than two vertices. If G1 Ç G2 =(V , E1ÇE2 is not a connected graph, then the graph
G1 È G2 = ( V , E1 È E2 )
(a) cannot have a cut vertex
(b) must have a cycle
(c) must have a cut-edge (bridge)
(d) has chromatic number strictly greater than those of G1 and G2 and
82. Let A[1,…,n] be an array storing a bit (1 or 0) at each location, and f(m) is a function whose time complexity is q(m). Consider the following program fragment written in a C like language:
counter = 0;
for (i =1; i < = n; i++)
{if (a[i] == 1) counter++;
else {f (counter); counter = 0;)
}
The complexity of this program fragment is
(a) W( n2) (b) W (nlogn) and O (n2)
(c) q (n) (d) o(n)
83. The time complexity of the following C function is (assume n > 0)
int recursive (int n) {
if (n == 1)
return (1);
else
return (recursive (n-1) + recursive (n-1);
}
(a) O(n) (b) O(nlog n) (c) O n (d) O(2n)
84. The recurrence equation
T (1) = 1
T (n) = 2T (n-1) + n, n ³ 2
evaluates to
(a) 2n+1 - n - 2 (b) 2n - n (c) 2n+1 -2n - 2 (d) 2n + n
85. A program takes as input a balanced binary search tree with n leaf nodes and computes the value of a function g(x) for each node x. If the cost of computing g(x) is min(number of leaf-nodes in left-subtree of x, number of leaf-nodes in right-subtree of x) then the worst-case time complexity of the program is
(a) €(n) (b) € (n log n) (c) € (n2) (d) € (n2 log n)
86. The following finite state machine accepts all those binary strings in which the number of 1's and 0's are respectively
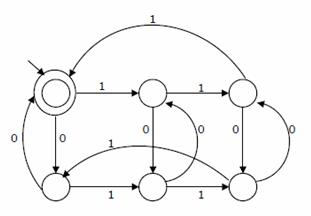
(a) divisible by 3 and 2 (b) odd and even
(c) even and odd (d) divisible by 2 and 3
87. The language ,{ambncm+n |m,n ³ 1} is
(a) regular (b) context-free but not regular
(c) context sensitive but not context free (d) type-0 but not context sensitive
88. Consider the following grammar G:
S® bS|aA|b
A® bA|aB
B® bB |aS| a
Let Na (w)Nb (w)and denote the number of a's and b's in a string respectively.
(a) {w|Na(w) > 3Nb (w)} (b) {w|Nb(w) > 3Na (w)}
(c) {w|Na(w) =3k, k Î {0,1,2,.....}} (d) {w|Nb(w) =3k, k Î {0,1,2,.....}}
89. L1 is a recursively enumerable language over å. An algorithm A effectively enumerates its words as w1, w2, w3, … define another language L2 over å È {#} as {wi # wj : wj Î L1, i < j}. Here # is a new symbol. Consider the following assertions.
S1 : L1 is recursive implies L2 is recursive
S2 : L2 is recursive implies L1 is recursive
Which of the following statements is true?
(a) Both S and S are true
(b) S1 is true but S2 is not necessarily true
(c) S2 is true but S1 is not necessarily true
(d) Neither is necessarily true
90. Choose the best matching between the programming styles in Group1 and their characteristics in Group 2.
Group 1 Group 2
P. Functional 1. Command-based, procedural
Q. Logic 2. Imperative, abstract data types
R. Object-oriented 3. Side-effect free, declarative, expression evaluation
S. Imperative 4. Declarative, clausal representation, theorem proving
(a) P - 2 Q - 3 R - 4 S - 1 (b) P - 4 Q - 3 R - 2 S - 1
(c) P - 3 Q - 4 R - 1 S - 2 (d) P - 3 Q - 4 R - 2 S - 1
0 comments:
Post a Comment
Comments : Read Them Or Add One to promote us